If you're searching for the 'Computer Programming Word Search' answers, you're in the right place. Here's where you'll find the complete answer key. It includes terms like data structures, control flow, functions, loops, arrays, and variables. Plus, you'll uncover details on algorithms, compilation, syntax, and comments. Keep exploring to deepen your understanding of programming essentials.
Key Takeaways
- Answer key provides solutions to word search puzzles in computer programming terminology.
- Helps identify and match programming-related words within the word search grid.
- Allows users to check correctness and completeness of their word search findings.
- Enhances learning by reinforcing programming concepts through word search activities.
- Aids in familiarizing with key terms, functions, loops, arrays, and variables in a fun and interactive way.
Key Terms
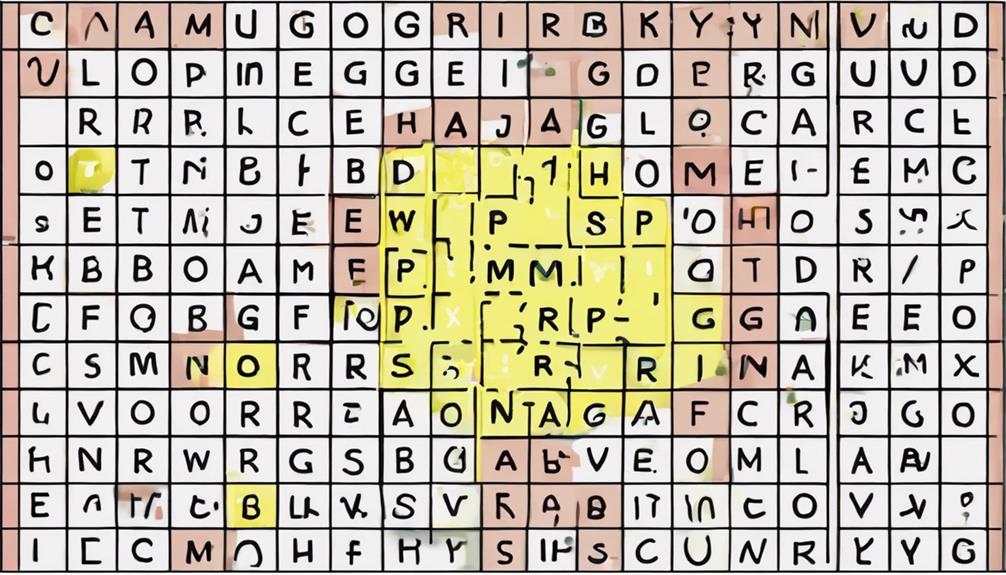
Let's explore the essential key terms used in computer programming. Two fundamental concepts that you'll frequently encounter are data structures and control flow.
Data structures refer to the different ways in which data can be organized and stored in a program. This includes arrays, linked lists, stacks, queues, and more. Understanding data structures is vital for efficient data manipulation and storage within a program.
Control flow, on the other hand, dictates the order in which the various parts of a program are executed. It includes concepts such as loops, conditional statements, and function calls.
Loops allow you to repeat a certain block of code multiple times, while conditional statements like if-else help in making decisions within the program. Function calls enable you to break down your code into smaller, reusable parts for better organization and readability.
Functions
Understanding functions is essential in computer programming as they allow you to encapsulate reusable blocks of code for better organization and efficiency. Functions in programming serve as building blocks, enabling you to break down complex problems into smaller, more manageable parts.
Recursion in functions is a powerful concept where a function calls itself either directly or indirectly. This technique is often used in scenarios where a problem can be divided into smaller subproblems that are of the same type as the original problem.
When analyzing functions, considering their time complexity is vital. Time complexity analysis of functions helps you understand how the runtime of a function grows concerning the size of its input. This analysis provides insights into the efficiency of your code and helps you optimize it for better performance.
Loops
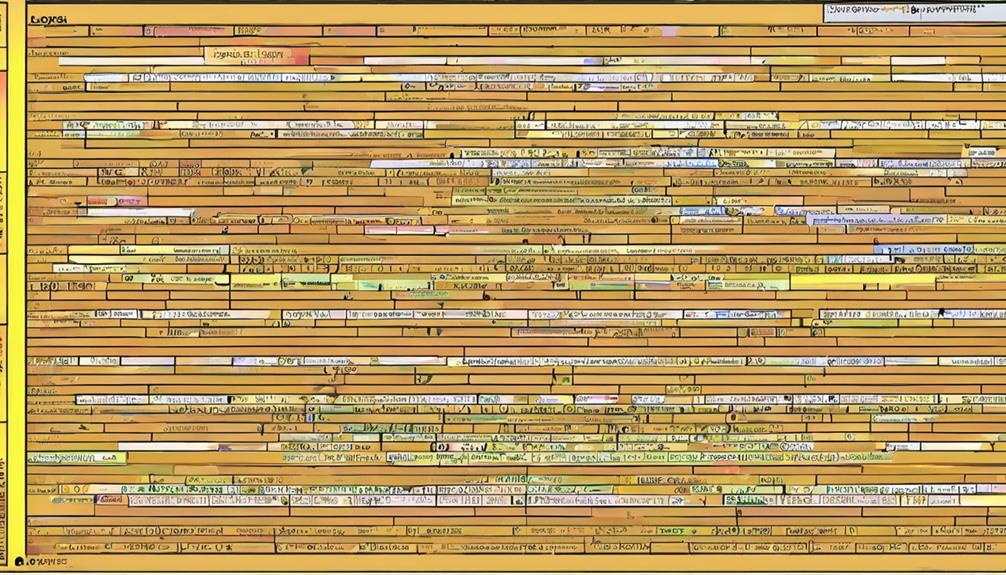
Mastering loops is vital in programming as they allow you to execute a block of code repeatedly based on a condition. When working with loops, it's important to take loop optimization techniques into account to enhance efficiency. Loop optimization involves strategies to improve loop iteration complexity, reducing the time and resources needed for the loop to complete.
Additionally, loop performance analysis plays a significant role in determining the effectiveness of your code. By analyzing the performance of loops, you can identify bottlenecks and areas for improvement, leading to a more streamlined and faster program.
Moreover, loop parallelization techniques can be employed to execute iterations concurrently, leveraging the power of multi-core processors for enhanced performance. By parallelizing loops, you can distribute the workload across multiple threads, increasing efficiency and speeding up the overall execution of your code.
Remember to always aim for efficient and optimized loops to make sure your programs run smoothly and effectively.
Arrays
Arrays store multiple elements of the same data type in a contiguous memory location, allowing for efficient access and manipulation of data in programming. When working with arrays, you can perform various array manipulation operations, such as sorting, searching, and inserting elements. These operations are essential for organizing and processing data effectively.
Array Manipulation
Here is a basic example of a 3×5 array:
Column 1 | Column 2 | Column 3 |
---|---|---|
Row 1 | Row 1 | Row 1 |
Row 2 | Row 2 | Row 2 |
Row 3 | Row 3 | Row 3 |
Row 4 | Row 4 | Row 4 |
Row 5 | Row 5 | Row 5 |
Multi-dimensional arrays go beyond one-dimensional arrays by organizing elements in multiple dimensions. They are beneficial when dealing with complex data structures that require more than one index to access elements efficiently. Understanding array manipulation and multi-dimensional arrays is fundamental in mastering programming concepts.
Variables
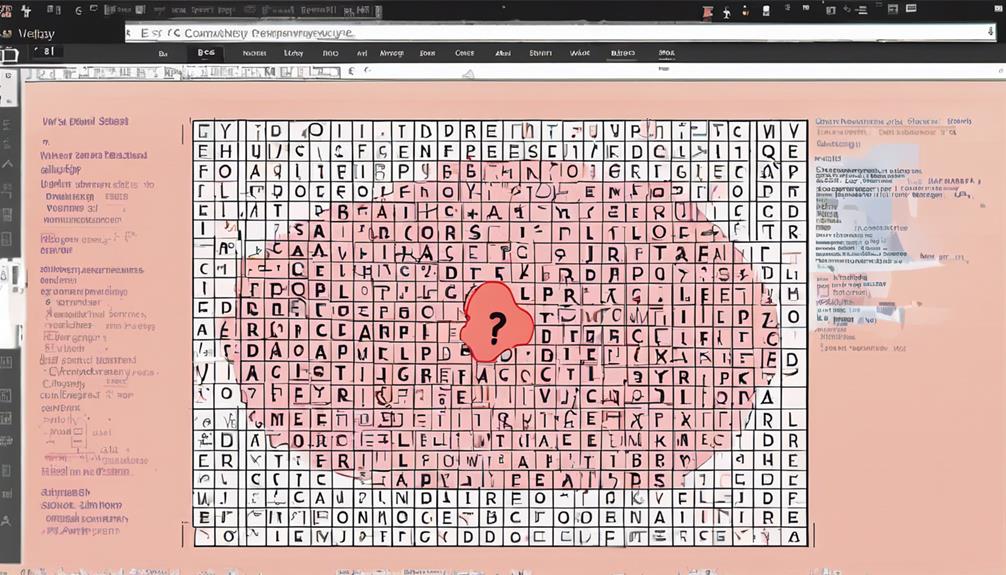
In programming, variables act as placeholders for storing data that can be changed or manipulated during the execution of a program.
When working with variables, understanding data types and manipulation is essential. Data types define the kind of data a variable can hold, such as integers, strings, or booleans. Manipulation involves operations like assigning new values, performing calculations, or concatenating strings to variables.
Scope and memory allocation are also important concepts related to variables. Scope determines the accessibility and visibility of variables within different parts of the program. Variables can have local scope, limited to specific functions or blocks of code, or global scope, accessible throughout the program.
Memory allocation refers to the process of reserving space in the computer's memory for storing variable data. Proper memory management ensures efficient use of resources and prevents memory leaks.
Debugging
To effectively debug a program, you must systematically identify and resolve any errors in the code. When faced with error messages, it's important to understand what they're indicating. Begin by carefully reading the error message to pinpoint the issue.
Common troubleshooting techniques involve checking for syntax errors, examining variable values during runtime, and using debugging tools provided by your programming environment.
Implementing best practices for efficient debugging can save you time and frustration. Start by breaking down the code into smaller sections to isolate the problem area. Utilize print statements or logging to track the flow of execution and identify where the code is deviating from the expected behavior.
Additionally, consider using version control systems to track changes and revert to a working state if needed.
Algorithm
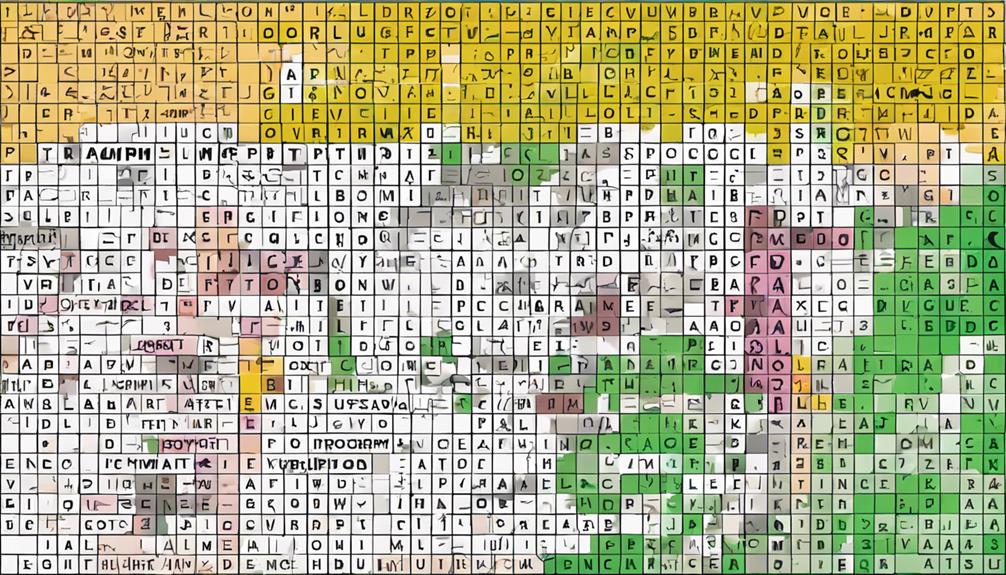
Understanding algorithms is important for developing efficient and effective computer programs. When designing algorithms, you have the option to represent them using pseudocode or flowcharts. Pseudocode provides a high-level description of the algorithm's logic without getting into specific syntax, while flowcharts visually depict the steps in a more graphical manner.
In addition to choosing the right representation, analyzing algorithm efficiency is key. This involves evaluating how well an algorithm performs in terms of time and space complexity. By evaluating algorithm efficiency, you can determine the most effective solution for a given problem. Techniques such as Big O notation help in quantifying the performance of algorithms as input sizes grow.
When comparing pseudocode and flowcharts, consider the readability and ease of understanding for yourself and other developers. Evaluating algorithm efficiency ensures that your programs run smoothly and effectively, saving time and resources in the development process.
Compilation
You often compile computer programs to translate source code into executable files. Compilation is an important step in software development.
When you write code in coding languages like C++, Java, or Python, the code is in human-readable format. However, computers can't directly understand this code. That's where compilation comes in.
During the compilation process, the source code is converted into machine code that the computer can execute. This machine code is what enables the software to run smoothly on your device.
Compilers play a significant role in this process by checking for errors and translating the code efficiently.
Software development heavily relies on compilation to make sure that the code you write functions correctly and efficiently. Without compilation, coding languages would be incomprehensible to computers, making it impossible to create the vast array of software we use daily.
Syntax
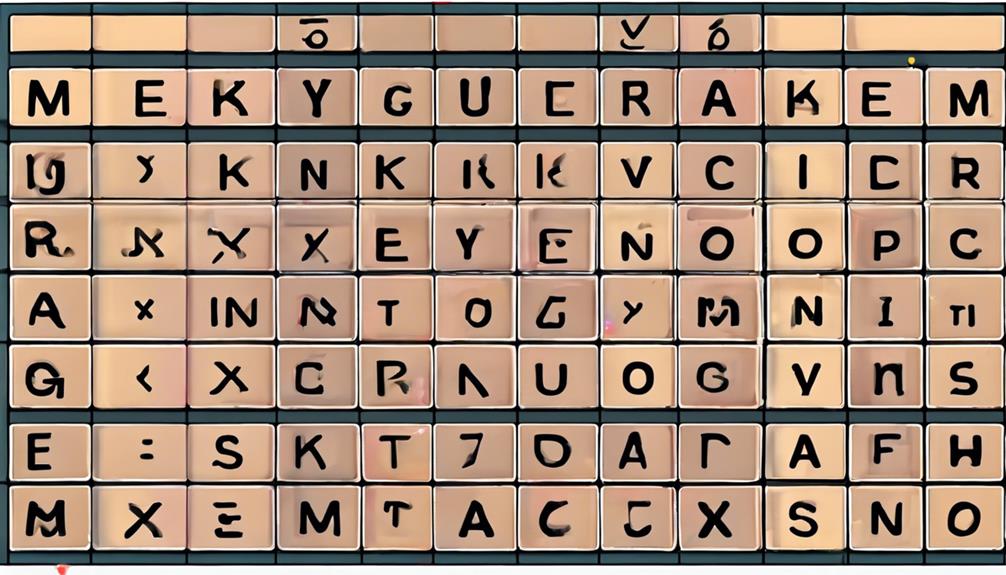
When writing computer programs, having a good grasp of syntax is key to ensuring your code functions as intended. Syntax refers to the rules that dictate how programming languages are structured and how commands must be written for the computer to understand them.
Understanding syntax is essential for correctly defining variables, using control structures like loops and conditionals, and implementing functions or methods.
Data structures are essential components in programming that allow you to organize and manipulate data efficiently. Arrays, lists, stacks, and queues are examples of data structures that have specific syntax for declaration and manipulation.
Mastery of these syntax rules enables you to work with complex data structures effectively, optimizing the performance of your programs.
Error handling is another critical aspect of programming syntax. Properly handling errors involves using syntax like try-catch blocks in languages such as Java or using if-else statements to manage unexpected scenarios.
Comments
Effective use of comments in your code enhances readability and understanding for yourself and others who may work on the program. Comments play an important role in explaining complex sections of code, documenting key algorithms, and providing insights into the logic behind certain decisions.
When it comes to collaboration, well-commented code allows team members to grasp the purpose of functions or classes quickly, speeding up development and troubleshooting processes.
To write effective comments, consider providing context instead of just describing what the code does. Explain why certain approaches were chosen or mention any potential pitfalls to watch out for. Be concise yet informative, using clear language that adds value to the reader's understanding.
While comments are essential, avoid over-commenting, as redundant comments can clutter the code and make it harder to read. Aim for a balance between code clarity and comment usefulness, ensuring that your comments enhance the overall comprehension of the codebase without being excessive.
Conclusion
To sum up, you have successfully completed the computer programming word search and have gained a better understanding of key terms such as functions, loops, arrays, variables, and algorithms.
Remember to practice writing code, understand compilation and syntax, and use comments to explain your work.
Keep up the great work in your programming journey!